How to use Python filter() easy. Tutorial and examples
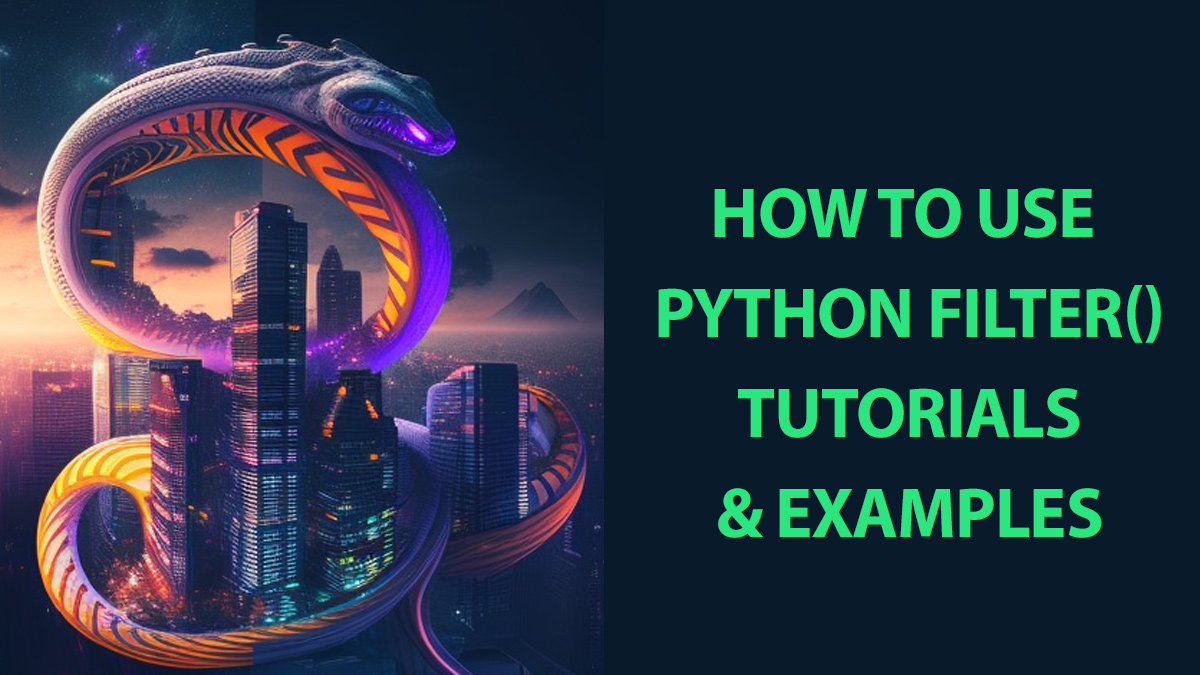
The filter() function in Python is a built-in function that allows you to create a new iterator from an existing iterable (such as a list or a dictionary) that will efficiently filter out the elements using a function that you provide. For example, if you have a list of numbers and you want to keep only the even ones, you can use filter() with a function that checks if a number is divisible by 2.
The basic syntax of the filter()
function is as follows:
filter(function, iterable)
Here, function
is the function that will be applied to each element of the iterable
object. The filter()
function returns an iterator of the elements in the iterable
object for which the function
returns True
.
Here are some real-world examples of how to use the filter()
function in Python:
# filter out odd numbers from a list of integers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6, 8, 10]
# filter out strings that are shorter than 5 characters from a list of strings
words = ["apple", "banana", "pear", "orange", "grape", "kiwi"]
long_words = list(filter(lambda x: len(x) >= 5, words))
print(long_words) # Output: ["apple", "banana", "orange"]
# filter out items from a dictionary whose values are less than 50
items = {"item1": 45, "item2": 60, "item3": 30, "item4": 75}
filtered_items = dict(filter(lambda x: x[1] >= 50, items.items()))
print(filtered_items) # Output: {"item2": 60, "item4": 75}
# filter out people who are not adults from a list of Person objects
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
people = [Person("Alice", 25), Person("Bob", 17), Person("Charlie", 30), Person("David", 12)]
adults = list(filter(lambda x: x.age >= 18, people))
for person in adults:
print(person.name) # Output: "Alice", "Charlie"
# filter out tuples from a list where the sum of the elements is less than 10
tuples = [(1, 2), (3, 4), (5, 6), (7, 8), (9, 10)]
filtered_tuples = list(filter(lambda x: sum(x) >= 10, tuples))
print(filtered_tuples) # Output: [(5, 6), (7, 8), (9, 10)]
# filter out dictionaries from a list where the value of the 'age' key is less than 30
people = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 17},
{"name": "Charlie", "age": 30},
{"name": "David", "age": 12}
]
filtered_people = list(filter(lambda x: x['age'] >= 30, people))
print(filtered_people) # Output: [{"name": "Charlie", "age": 30}]
# filter out dictionaries from a list where the value of the 'age' key is less than 30
people = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 17},
{"name": "Charlie", "age": 30},
{"name": "David", "age": 12}
]
filtered_people = list(filter(lambda x: x['age'] >= 30, people))
print(filtered_people) # Output: [{"name": "Charlie", "age": 30}]
# filter out numbers from a list that are not divisible by 3
numbers = [2, 3, 6, 8, 9, 12, 15, 17, 18, 21]
filtered_numbers = list(filter(lambda x: x % 3 == 0, numbers))
print(filtered_numbers) # Output: [3, 6, 9, 12, 15, 18, 21]
Here are some examples of how to use filter() with re.match():
- To filter a list of strings that start with ‘x’, you can use:
import re
lst = ['xray', 'xyz', 'apple', 'xerox']
result = list(filter(lambda x: re.match('x', x), lst))
print(result)
# ['xray', 'xyz', 'xerox']
import re
# filter out strings from a list that start with a lowercase letter
words = ["apple", "Banana", "pear", "Orange", "grape", "kiwi"]
filtered_words = list(filter(lambda x: re.match('^[a-z]', x), words))
print(filtered_words) # Output: ["apple", "pear", "grape", "kiwi"]
import re
# filter out filenames from a list that do not have the extension '.txt'
filenames = ["file1.txt", "file2.csv", "file3.txt", "file4.py"]
filtered_filenames = list(filter(lambda x: re.match('.+\.txt$', x), filenames))
print(filtered_filenames) # Output: ["file1.txt", "file3.txt"]
import re
# filter out email addresses from a list that do not have a '.com' domain
emails = ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
filtered_emails = list(filter(lambda x: re.match('.+@.+\.(com|org|net)$', x), emails))
print(filtered_emails) # Output: ["[email protected]", "[email protected]", "[email protected]"]
These examples demonstrate how you can use filter()
in combination with re.match()
to filter out elements from iterable objects based on regular expression patterns. Note that re.match()
returns a match object if the pattern matches the beginning of the string, so you can use it in a lambda
function within filter()
to apply the pattern to each element of the iterable.
The filter() function in Python is a built-in function that allows you to create a new iterator from an existing iterable (such as a list or a dictionary) that will efficiently filter out the elements using a function that you provide. For example, if you have a list of numbers and you want to keep…